Bash: parsing command line arguments with getopt
In this example we have a function that can optionally! accept the following parameters:
-v -d --debug --name VALUE
The call to
Then we have a whole while loop to actually take out the values from the list on the comand line
and to assign the appropriate values to variables.
Either 1 to the variables representing the switches, or the actual value passed to the --name option.
At the end we need to call this function with the args $0 "$@" expression.
examples/shell/cli.sh
I used to have this, in the above code:
This is I think the shell-style meaning:
"Either the previous command is successful (exit 0) or `||` do the block (echo and exit)".
Later I realized using `if` would make it much more readable so I changed it to:
They both do the same.
#!/bin/bash
function args()
{
options=$(getopt -o dv --long debug --long name: -- "$@")
if [ $? -ne 0 ]; then
echo "Incorrect option provided"
exit 1
fi
eval set -- "$options"
while true; do
case "$1" in
-v)
VERBOSE=1
;;
-d)
DEBUG=1
;;
--debug)
DEBUG=1
;;
--name)
shift; # The arg is next in position args
NAME=$1
;;
--)
shift
break
;;
esac
shift
done
}
args $0 "$@"
echo $NAME
echo $DEBUG
echo $VERBOSE
An example calling it
$ ./cli.sh --name "Foo Bar" --debug -v
Foo Bar
1
1
$ ./cli.sh --wrong
getopt: unrecognized option '--wrong'
Incorrect option provided
If condition in bash
[ $? -eq 0 ] || {
echo "Incorrect option provided"
exit 1
}
if [ $? -ne 0 ]; then
echo "Incorrect option provided"
exit 1
fi
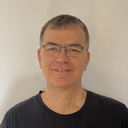
Published on 2019-06-17