Show dates in the timezone of the reader using JavaScript
I am running live online events that people can join from anywhere in the world if they know when the events start. Computing and showing the date and time in every possible timezone is exhausting and would be probably confusing to the reader. The best is to show the scheduled date and time in their local time-zone.
Encouraged by the posts of Mark Gardner I wanted to implement it using JavaScript only. This is what I got:
In the first example we have a date string in the mydate variable and then we use the toLocaleString method with various dateStyle options and toLocaleDateString options to show the date in different formats. You can click on the try link below the example to see the code running in a separate tab.
examples/javascript/date.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=yes"> <title>Date</title> </head> <body> <div id="default"></div> <div id="long"></div> <div id="medium"></div> <div id="short"></div> <div id="special-long"></div> <div id="special-short"></div> </body> </html> <script> const mydate = "2021-04-13T19:00:00-04:00"; const date = new Date(mydate); document.getElementById("default").innerHTML = date; document.getElementById("long").innerHTML = date.toLocaleString( [], {dateStyle: "long"}) document.getElementById("medium").innerHTML = date.toLocaleString( [], {dateStyle: "medium"}) document.getElementById("short").innerHTML = date.toLocaleString( [], {dateStyle: "short"}) document.getElementById("special-long").innerHTML = date.toLocaleDateString( [], { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', timeZoneName: 'long' }) document.getElementById("special-short").innerHTML = date.toLocaleDateString( [], { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', timeZoneName: 'short' }) </script>Try!
See the list of options of toLocalString
A more real-world version
In the second example you can see a more real-world version. The date string is in an attribute of a div element. It could have been added there manually or by some back-end application with a template.
Then there is a function that check if there is an HTML element with the "localdate" id. Takes the attribute from it and uses the same techniques as above to set the date in a nice format. (Use the try link to see it working.
examples/javascript/localdate.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=yes"> <title>Date</title> </head> <body> <div id="localdate" x-schedule="2021-04-13T19:00:00-04:00"></div> </body> </html> <script> function set_local_date() { // Assume a date format of "2021-04-13T19:00:00+03:00"; // Display time in localtime of the browser. const obj = document.getElementById("localdate"); if (! obj) { return; } const mydate = obj.getAttribute("x-schedule"); const date = new Date(mydate); if (obj) { obj.innerHTML = date.toLocaleDateString( [], { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', timeZoneName: 'long' }); } } set_local_date(); </script>Try!
Live events
Now it is your turn to check out our live events and join one.
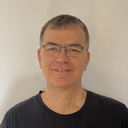
Published on 2021-05-07