Backend Python developer - Junior Python programmer
Many companies advertise job positions as Python programmer, or Backend developer. Sometimes Backend Python developer.
So what do you need to know in order to become one? What do you need to find a Junior level position with a title like that?
Non-Technical Skills
Though I think it would be very important to have a broad view of the product your company is building, in many cases, especially as a junior programmer, you will only focus on a small corner of the project. So as a junior programmer it is probably more important to be able to focus on specific tasks than to have a broad view.
Being able to work alone but also being a team-player is often part of the job description. On the one hand you need to be able to read documentation and find solutions by searching and you won't need to be micro-managed. On the other hand you can compromise if some other developer thinks a different solution is better.
Communication: The tech world is full of people who have a hard time communicating with each other. Being able to communicate will give you a big advantage. It is becoming more and more important to be able to communicate in writing, both in your own language and in English. Verbal communication skills are also useful, though as the companies become more and more distributed, writing will be probably more important.
Some job posts will mention that you need to be able to work under pressure. That sounds like a symptom of bad management. I would avoid such companies.
Python
Obviously you will need to know how to program in Python, though at what level you need to know is not clearly defined and it depends on the specific position.
You will need to know the basic data types in Python (int, float, str, bool).
You will need to be familiar with the data structures of Python (list, dictionary, tuple) and you'll need to be able to handle multiple dimensions of these data structures. (e.g. list of dicts of dicts).
Other structures such as set, queue, stack and special versions of dictionaries are also useful, but they might not be prerequisites to getting an entry level job.
Functions and modules are considered basics, though I think you don't necessarily need to understand packages.
Knowing some of the standard libraries of Python is a requirement (e.g. os, sys, shutil, argparse, logging) though of course "knowing" here means that you are aware of them and you know some of their methods. Not that you know them in full detail.
Knowing regular expressions is usually not a requirement, but they can give you super-powers, so I'd recommend you learn them.
Exception handling is probably a requirement.
Understanding OOP - Object Oriented Programming is probably a requirement everywhere.
One area that is often overlooked is to know how to write tests in Python. Often referred to as unit tests, though in the case of Python this is a bit confusing as there is also a library called unittest. The idea is to know the concept so it does not really matter if you use the unittest module or the pytest module.
The first 15-20 chapters of my Python programming course covers these topics.
Git (Version Control)
As a programmer you will have to be familiar with at least one Version Control system. These days Git is the most popular VCS together with one of the cloud-based Git hosting services such as GitHub, GitLab, or BitBucket.
Bug tracking system
Most companies use some kind of a bug-tracking system, so you need to be familiar at least with the concept. The various Git-hosting services each have their own system so you can learn and practice.
SQL / No-SQL
Knowing SQL and in general Relational Databases can be useful though many back-end Python programmers never touch a database.
No-SQL databases can also be useful but are also only needed in a subset of the jobs.
API - JSON
Many applications either provide APIs or use other 3rd-party APIs. APIs usually communicate using JSON. Just as with databases, being familiar with APIs can be useful in many positions.
Web frameworks: Flask, Django...
A large subset of backend developers write web applications. They need to know the appropriate web framework used at their company. There are many Python-based web frameworks, but the most popular ones are Django and Flask. Learn at least one of them to increase your chances to become a good backend developer. Hint: Flask is much easier to learn.
CI - Continuous Integration
You should be familiar with the concept. Extra value if you know how to use one of the cloud-based CI systems. (e.g. Travis CI)
Containers - Docker
In many companies there will be a "DevOps engineer", who will deal with Docker, but if you know it you will have a nice advantage.
Other
There are many other things you'll need to learn, many of those are job-specific.
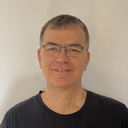
Published on 2020-03-11