Groovy: import and use functions from another file
Code reuse is an important part of programming. The smallest possible way is to create functions and call them several times in the same code. The next step is to have a set of functions in a file and use those functions in several other files.
In these examples we see how to do that with simple functions and methods of classes.
Importing function
In this example we have a very simple "library" file with a simple function:
examples/groovy/function_tools.gvy
def greet() { println "Hello World!" }
Next to it there is another file in which we load the former file into memory and then call it.
examples/groovy/function_script.gvy
GroovyShell shell = new GroovyShell() def tools = shell.parse(new File('function_tools.gvy')) tools.greet()
We can then run
groovy function_script.gvy
In the code we gave the path to the library so in this version it needs to be next to the code loading it.
Importing class methods
This is the class:
examples/groovy/class_tools.gvy
class Tools { def greet() { println "Hello World!" } }
This is the script:
examples/groovy/class_script.gvy
def tools = new GroovyScriptEngine( '.' ).with { loadScriptByName( 'class_tools.gvy' ) } this.metaClass.mixin tools greet()
Importing class methods
examples/groovy/a/main.groovy
import tools t = new tools() t.hello() // Hello World // this also works: new tools().hello() // Hello World
examples/groovy/a/tools.groovy
class tools { def hello() { println("Hello World") } }
Import class from a subdirectory
examples/groovy/b/main.groovy
import tools.other new other().hi()
examples/groovy/b/tools/other.groovy
package tools class other { def hi() { println("Hello Again") } }
Import static methods from a class
This is probably the version most similar to importing individual functions.
examples/groovy/d/main.groovy
import static tools.hello as hi hi()
examples/groovy/d/tools.groovy
class tools { static hello() { println("Hello World") } }
Import static methods from a class from a subdirectory
examples/groovy/e/main.groovy
import static some.other.Tools.hello as hi hi()
examples/groovy/e/some/other/Tools.groovy
package some.other class Tools { static hello() { println("Hello World") } }
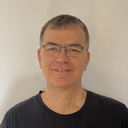
Published on 2018-09-13