AngularJS - first binding
Now that we have created our very first expressions in AngularJS, its time to make another step, this time with something much more interesting. We are going to connect an input field with an expression that will automatically display whatever we type in.
Minimal Hello User
The Hello World examples are usually quite boring as they are one-way. Just display some string that was part of the code. In this example we have an input element in which we declare the ng-model with a value name.
<input ng-model="name">
Once we do that we can use the name attribute in Angular expressions: {{ name }} for example in order to show the content:
examples/angular/minimal_hello_user.html
<script src="angular.min.js"></script> <div ng-app> <input ng-model="name"> <h1>Hello, {{name}}</h1> </div>Try!
If you open this example, you'll see an input box. As you type in the input box the text you type in will also appear after the word Hello.
With this we see how can we bind input elements to attributes of AngularJS that can be used in expressions.
Full Hello User example
The above was probably the smallest possible example using data binding in AngularJS. A full, or at least "fuller" example can be found here:
examples/angular/hello_user.html
<!DOCTYPE html> <html ng-app> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=yes"> <title></title> <script src="angular.min.js"></script> </head> <body> <input ng-model="name" type="text" placeholder="Your name please"> <h1>Hello, {{name}}</h1> </body> </html>Try!
In this version we have a "real" HTML 5 page, the ng-app marks the whole html file to be our Angular Application, and the input element is also better described with type and a placeholder to give a hint to the user what to do with the HTML form.
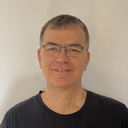
Published on 2015-07-24