Introduction to JavaScript - basic output
While learning about Node.js, JQuery, or Angular.js might be fun, I think it is important to have some background, and to know how to use plain JavaScript. This series of articles will provide an introduction to JavaScript.
Before we get to the syntax, first let's understand where can we run JavaScript.
Traditionally JavaScript was used inside web browsers such as Mozilla Firefox, Internet Explorer, Chrome, Opera, or Safari. The author would include some JavaScript code in the HTML page the user receives when she visits a web site. That JavaScript code would run in the browser (what we call "client side", as opposed to running on the web server which is called "server side").
In recent years people have started to put JavaScript code on the server as well. Probably the most well know environment to run JavaScript on the server is Node.js, but there are others. For example io.js which started as a Node.js fork.
We can distinguish 3 major parts of what we usually refer to as "JavaScript".
- The language itself. This is fairly standard among the various environments, both in the various browsers and in the various server-side environments.
- The DOM API - how the language can interact with the various parts of a web page while in the browser. While in this respect the various browsers are getting closer to each other they still differ. Several libraries, most prominently JQuery, is trying to provide a unified API.
- The server API (or just API) provided by Node.js or one of the other server-side systems.
In this series of articles we'll see all 3 major components.
Let's start with a few simple examples we can run in a browser. It is probably the easiest to get started this way, as these examples only require you to have a browser (and if you are reading this, then you probably have one), and a text editor.
Editor or IDE
Any text editor can be used.
On MS Windows you can even use the built-in plain Notepad, but I'd recommend something more powerful. You can download Notepad++ which is very similar to Notepad, but with tons of extra features, or get Aptana Studio. The latter has a steeper learning curve, so you might want to start with the more simple tool.
Embed or include
You can either embed the JavaScript code directly inside the HTML file, or you can put a line in the HTML file that will include the external JavaScript file. In most cases the latter is recommended, but for our first examples, in order to make the whole thing work in a single file, we'll embed the JavaScript code inside some HTML.
In order to do that we add the <script> opening and </script> closing tags. Between the two we write our JavaScript code.
Input Output
The very first thing we need to learn is how to interact with the JavaScript code running in the browse. There are a number of way JavaScript can display text for the user (output). The most simple one is by using the alert function:
alert
This will show a pop-up in the browser with the text. (You can click on Try! that will open the specific script in a separate window.) The alert() function is actually rarely used, but it is an easy way to show the use of JavaScript.
examples/js/alert.html
<script language="javascript"> alert("Hello World"); </script>Try!
If you'd like to try it yourself, open your editor and create a file with .html extension (for example hello.html) and put the above code in the file. Then switch to your browser and open the file with the browser. (Most browsers will let you do that using the File/Open File menu option.)
document.write
examples/js/document_write.html
First line <script> document.write("<h1>Hello World</h1>"); </script> Last lineTry!
In this example we have some text (First line), then the JavaScript code, and then some more text (Last line). The JavaScript code uses the document.write function to change the content of the page. It will embed the html snippet <h1>Hello World</h1> after the "First line", but before the "Last line".
This function was often used when one wanted to change what's shown. Today, there are some more advanced techniques.
console.log
Finally let's see how developers usually print out debugging information.
examples/js/console.html
<script> console.log("Hello World"); </script>Try!
Most of the web browsers provide what is called a "JavaScript console". It is an additional window which is normally not visible, where the browser can print out warnings and errors generated by the execution of the JavaScript code. (E.g. if there is a syntax error in the code.) The developer can also print information to this console using the console.log() call.
In order to see the console you'll need to open it.
If you happen to use Chrome on OSX you can open the console using: Command-Option-J.
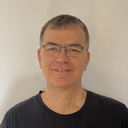
Published on 2015-02-09