Bash prompt showing git status
This code snippet can be included un the ~/.bash_profile It will change the Linux shell prompt to indicate the name of the current branch (if the current working directory is inside a git repository). It will also use color-code to indicate if there are non-committed files in the current repository.
Red for when there are non-committed files, yellow when everything is committed, but some changes have not been pushed to remote yet, and green when the directory is clean.
Of course it works on the current branch so if you have unpushed commits in other branches this won't indicate it.
examples/bash_git_prompt.sh
# Bash: when in a git repository, show the current branch, indicate if the working directory is clean or not. function __set_my_prompt { local RED="\033[0;31m" local GREEN="\033[0;32m" local NOCOLOR="\033[0m" local YELLOW="\033[0;33m" local BLACK="\033[0;30m" local git_modified_color="\[${GREEN}\]" local git_status=$(git status 2>/dev/null | grep "Your branch is ahead" 2>/dev/null) if [ "$git_status" != "" ] then git_modified_color="\[${YELLOW}\]" fi local git_status=$(git status --porcelain 2>/dev/null) if [ "$git_status" != "" ] then git_modified_color="\[${RED}\]" fi local git_branch=$(git branch --show-current 2>/dev/null) if [ "$git_branch" != "" ]; then git_branch="($git_modified_color$git_branch\[${BLACK}\]) " fi PS1="\[${BLACK}\]\u@\h \w $git_branch$\[${NOCOLOR}\] " } PROMPT_COMMAND='__set_my_prompt'
Feel free to reuse any part of this code.
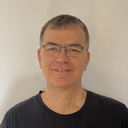
Published on 2021-06-21