Programming Exercises
When learning a programming language, the most important thing is to practice. Here are some exercises you can do. For each exercise, as time permits, I am going to add instructions specific to some programming languages, but these exercises can be solved in almost any other programming language.
Once you feel comfortable with the exercises you can pick one of the more complex projects as well.
Introduction
Scalars
- Calculate Area of Rectangular (Standard I/O, if)
- Number guessing game (random, prompt, if)
- Create calculator
Files
- Sum of numbers in a file (open, loop +=)
- Analyze Apache log file (count localhost vs others, index, substr)
- Add more statistics
- Write report to file
Lists and Arrays
- Color selector
- Add numbers taken from CSV file
- Improve the color selector
- Improve number guessing game
- Count digits
- Sort based on secondary condition
- Display unique rows of a file
- Sort mixed strings
- Queue at the Doctor
- Check if number is prime
- Reverse Polish Calculator
Hashes, Dictionaries
- Count words in a file
- Parse HTTP GET parameters
- Sort scores
- Analyze Apache log file (count all the hits from all the clients)
- Parse file with variable width fields
Functions and Subroutines
Regular Expressions, Regexes
- Regex Exercises - part 1
- Match numbers with regex
- Match hexa, octal, binary numbers
- Match Roman numbers
- Split file path with regex
- Sort SNMP numbers
- Parse hours log file and create time report
- Parse INI or Config files.
Linux
Shell
3rd party libraries
- Install Module from CPAN/PyPi/Gems/etc.
Simple applications
- Counter
- Exercise: Implement the wc command of Linux/Unix (word count)
- Traverse directory implementing du
- One dimensional spacefight (aka. The spaceship operator)
- MasterMind
Mix
- Send plain text e-mail
- Send HTML e-mail
- Send e-mail with attachments
- Compare Wikipedia translations
- RSS/Atom to e-mail
Web development
Web crawlers
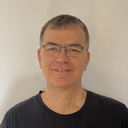
Published on 2015-10-14