Exercise: Implement Reverse Polish Calculator
Implement Reverse Polish Calculator
We are used to write calculations using the infix notation where the operator is between the two operands. e.g. 3 + 4 * 7. In order to implement a calculator that knows how to calculate this one needs to implement the order of operations.
In the Reverse Polish Notation the operator comes after the two operands. e.g. 3 4 + instead of 3 + 4.
In this notiation there are no preferences between operators.
The above expression can be written in RPN as:
3 4 7 * + =
The task is to implement RPN in your favorite language.
Python
In order to make it easer for you I've prepared a module that implements the low-level calculations.
examples/python/calc.py
def calc(a, op, b): ''' from calc import calc calc(2, '+', 3) ''' if op == '+': return a + b if op == '*': return a * b if op == '-': return a - b if op == '/': return a / b raise Exception("Operator '{}' not supported".format(op)) def test_calc(): assert calc(2, '+', 3) == 5 assert calc(2, '*', 3) == 6 assert calc(8, '-', 3) == 5 assert calc(8, '/', 2) == 4 import pytest with pytest.raises(Exception) as exinfo: calc(2, '**', 3) assert exinfo.type == Exception assert str(exinfo.value) == "Operator '**' not supported" # To test this module, run pytest calc.py
Solutions
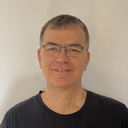
Published on 2018-04-21