Crystal: Swap Nibbles
In this weekly challenge the task was to swap the lower and upper 4 bits (nibbles) of an integer.
This is my implementation in the Crystal language.
At first I thought to convert the number to its binary representation and do the swapping there, but then I fugured it is just a simple mathematical operation.
- % is modulo
- // is integer division
Below the function I included the code to iterate over the range between 0 and 255 (inclusive) and for each iteration print the binary representation (padded with 0s) and the decimal representation of the original number followed by the decimal representation of the nibble-swapped number.
examples/crystal/swap_nibbles.cr
def swap_nibbles(n) return 16 * (n % 16) + (n // 16) end #puts swap(101) #puts swap(18) (0..255).each {|num| puts "%08b %3s %3s" % {num, num, swap_nibbles(num)} }
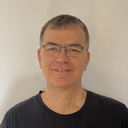
Published on 2021-07-02