Counter Example: Improved Vanilla JavaScript
This code is part of the counter example project. It is an impoved version of the Vanilla JavaScript counter I posted a while ago. This version was posted by Jon Randy as a reply to my post on dev.to.
examples/javascript/improved-vanilla-javascript-counter.html
<form> <input id="counter" type="button" value="0"> <input type="reset"> </form> <script> const form = document.forms[0] const increment = () => console.log(++form.counter.value) const reset = () => form.counter.value = 0 form.addEventListener('reset', reset) form.counter.addEventListener('click', increment) </script>Try!
A few notes as I am trying to understand this example:
- The value attribute of an input element of type="button" is the value shown by the browser on the button.
- I am still not used to the fat-arrow notation of creating anonymous functions in JavaScript that are then assigned to a variable. () => code is a function definition.
It is indeed a very nice use of the various default capabilities of HTML and JavaScript.
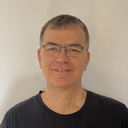
Published on 2023-01-31