Python Functional Programming: Introduction
In this series we are going to take a look at Functional programming in Python.
I am not trying to convince you to write in Functional programming. I definitely don't want you to write everything in functional programming style. Partially because probably you cannot.
If you really want to explore pure functional programming then pick up one of the purely functional programming language.
Instead of that I'd like to show you that you are probably already using various features of Python that are more aligned with the functional programming paradigm.
I am going to show you various further tools to improve that.
Programming paradigms
There are a number of Programming paradigms that you might be familiar with.
On the Programming paradigms page of Wikipedia you will see one of the ways to categorize programming languages.
There is imperative programming and underneath there are procedural programming and Object-oriented programming.
Then there are functional, logic, and mathematical programming. Since I recorded that video people also added reactive programming to this list.
There are, of course, other ways to categorize programming paradigms.
Basically procedural programming is what we are used to: just writing one statement after the other.
Object Oriented programming is when you create classes and call methods.
These are both supported by Python so you can write in both paradigms in Python.
Then there is the Functional programming that is also partially supported by Python. That's what we are going to focus on.
One more to mention is the Declarative programming paradigm. The language using this paradigm that you are most familiar with is probably SQL.
What is Functional Programming?
Functional programming has several features:
immutability - Python has a number of data-type that are immutable. For example strings and tuples.
The problem with variable is that they change. Well, they name indicates that, but apparently it is also a source of a lot of bugs.
Instead of changing the content of the immutable data-types Python allows you to replace the content of a variable with the new value.
Separation of data and functions is quite the opposite to what Object Oriented programming says. In OOP you have a class that has both attributes (data) and methods (functionality).
Pure functions are function without side-effects that given the same input will always return the same result. (So no counter, no print).
First-class functions (you can assign function to another name and you can pass function to other functions and return them as well. We can also manipulate functions) You can do all this in Python.
Higher order functions a functions that either takes a function as a parameter or returns a function as a parameter.
In python you can write a function that will receive a function as its parameter and returns another function based on the one that was just passed in. Basically this is what decorators do in Python.
We are going to talk about such features of Python in the following episodes.
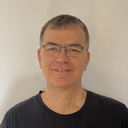
Published on 2022-11-25