Python: capturing, hiding, and reporting warnings
I am sure you have already seen warnings coming from some Python code. I espcially encountered warnings about using deprecated features.
This always raises threww questions:
How can I hide the warnings?
How can I capture, collect, and report the warnings?
How can you create my own warnings?
Generate warnings
examples/python/warn.py
import warnings def do_something(): warnings.warn("Some warning") def main(): print("before") do_something() print("after") main()
before warn.py:4: UserWarning: Some warning warnings.warn("Some warning") after
Capture and hide or print warnings
examples/python/warn_capture.py
import warnings def do_something(): warnings.warn("Some warning") warnings.warn("Other warning") def main(): print("before") with warnings.catch_warnings(record=True) as caught_warnings: warnings.simplefilter("always") do_something() for warn in caught_warnings: print("-----") print(f"warn: {warn.message}") print(warn.category) print(str(warn)) print("-----") print("after") main()
before ----- warn: Some warning <class 'UserWarning'> {message : UserWarning('Some warning'), category : 'UserWarning', filename : 'warn_capture.py', lineno : 4, line : None} ----- warn: Other warning <class 'UserWarning'> {message : UserWarning('Other warning'), category : 'UserWarning', filename : 'warn_capture.py', lineno : 5, line : None} ----- after
Log the warnings
examples/python/warn_to_logger.py
import warnings import logging def do_something(): warnings.warn("Some warning") def main(): logger = logging.getLogger('py.warnings') logging.captureWarnings(True) sh = logging.StreamHandler() sh.setLevel(logging.DEBUG) sh.setFormatter(logging.Formatter('%(asctime)s - %(levelname)-10s - %(message)s')) logger.addHandler(sh) logger.setLevel(logging.DEBUG) logger.info("before") do_something() logger.info("after") main()
2020-06-27 09:41:37,847 - INFO - before 2020-06-27 09:41:37,848 - WARNING - warn_to_logger.py:5: UserWarning: Some warning warnings.warn("Some warning") 2020-06-27 09:41:37,848 - INFO - after
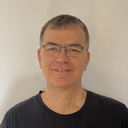
Published on 2020-06-27