Run code locally with Rexify
Usually you'll want your Rex commands to be executed on the remote server and thus the default of Rexify is to execute the rex command on the current remote server. However sometimes you'd want to have some Rex commands executed locally. There are two main ways to to that, either marking the task as no_ssh or marking a secion of the task as LOCAL.
Also remember that the perl code around the Rex commands are always executed locally on the management server.
This example demonstrates these cases:
examples/rex/local/Rexfile
use strict; use warnings; use 5.010; use Rex -feature => [qw(1.4 exec_autodie)]; use Sys::Hostname (); task 'remote', sub { sys_hostname(); run_hostname(); }; no_ssh task 'local', sub { sys_hostname(); run_hostname(); }; task 'partial', sub { sys_hostname(); run_hostname(); LOCAL { sys_hostname(); run_hostname(); }; }; sub run_hostname { my $hostname = run('hostname'); Rex::Logger::info("run('hostname'): $hostname"); } sub sys_hostname { my $hostname = Sys::Hostname::hostname(); Rex::Logger::info("Sys::Hostname::hostname() is always the local machine: $hostname"); } # vim: syntax=perl
In our example the hostname of the managemachine was code-maven and the name of the remote server was rex-host.
$ rex -H 104.236.89.4 -u root remote [2021-04-11 09:50:50] INFO - Running task remote on 104.236.89.4 [2021-04-11 09:50:54] INFO - Sys::Hostname::hostname() is always the local machine: code-maven [2021-04-11 09:50:55] INFO - run('hostname'): rex-host [2021-04-11 09:50:55] INFO - All tasks successful on all hosts
$ rex -H 104.236.89.4 -u root local [2021-04-11 09:51:11] INFO - Running task local on 104.236.89.4 [2021-04-11 09:51:11] INFO - Sys::Hostname::hostname() is always the local machine: code-maven [2021-04-11 09:51:11] INFO - run('hostname'): code-maven [2021-04-11 09:51:11] INFO - All tasks successful on all hosts
$ rex -H 104.236.89.4 -u root partial [2021-04-11 09:51:20] INFO - Running task partial on 104.236.89.4 [2021-04-11 09:51:24] INFO - Sys::Hostname::hostname() is always the local machine: code-maven [2021-04-11 09:51:24] INFO - run('hostname'): rex-host [2021-04-11 09:51:24] INFO - Sys::Hostname::hostname() is always the local machine: code-maven [2021-04-11 09:51:24] INFO - run('hostname'): code-maven [2021-04-11 09:51:24] INFO - All tasks successful on all hosts
See also: no_ssh
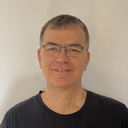
Published on 2021-04-11