Mobile swipe left, swipe right of HTML pages using vanilla JavaScript
At one point I wanted to add this feature to my slides but I later remove it as some of the pages needed to have horizontal scrolling and this code did not work well together with that. Maybe the only thing I need is to adjust some of the parameters. So check it out maybe by the time you read this, the swiping on my slides might work.
Anyway, if you have some HTML pages you might want to allow your users to move between pages, using JavaScript, but you don't want to rely any of the JavaScript libraries.
examples/javascript/swipe_vanilla.js
(function() { var min_horizontal_move = 30; var max_vertical_move = 30; var within_ms = 1000; var start_xPos; var start_yPos; var start_time; function touch_start(event) { start_xPos = event.touches[0].pageX; start_yPos = event.touches[0].pageY; start_time = new Date(); } function touch_end(event) { var end_xPos = event.changedTouches[0].pageX; var end_yPos = event.changedTouches[0].pageY; var end_time = new Date(); let move_x = end_xPos - start_xPos; let move_y = end_yPos - start_yPos; let elapsed_time = end_time - start_time; if (Math.abs(move_x) > min_horizontal_move && Math.abs(move_y) < max_vertical_move && elapsed_time < within_ms) { if (move_x < 0) { //alert("left"); } else { //alert("right"); } } } var content = document.getElementById("content"); content.addEventListener('touchstart', touch_start); content.addEventListener('touchend', touch_end); })();
- Wrap the whole area where you'd like the swipe to work in a div with an ID=content.
- Replace the two commented-out alert calls by function calls implementing the left-swipe and the right swipe actions.
- Adjust the 3 numbers to your liking.
- Done.
var min_horizontal_move = 30; var max_vertical_move = 30; var within_ms = 1000;
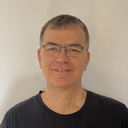
Published on 2020-07-23