Terraform
This post isn't really finished, but one day it might be useful.
infrastructure as code HashiCorp Terraform
examples/terraform/1/main.tf
provider "google" { project = "project-name-1234" region = "us-central1" } resource "google_compute_instance" "gabor" { name = "gabor-1" machine_type = "f1-micro" zone = "us-central1-a" boot_disk { initialize_params { image = "ubuntu-1804-lts" } } network_interface { subnetwork = "central1-resources" } }
terraform init terraform apply
examples/terraform/2/main.tf
provider "google" { project = "project-name-12345" region = "us-central1" } resource "google_compute_instance" "gabor" { name = "gabor-${count.index + 1}" machine_type = "f1-micro" zone = "us-central1-b" count = "2" labels = { owner = "gabor" group = "devops" } # tags = ["access-external"] boot_disk { initialize_params { image = "ubuntu-1804-lts" } } network_interface { subnetwork = "central1-resources" } scheduling { preemptible = false automatic_restart = false } }
examples/terraform/count/main.tf
vars { total = 1 } resource "google_compute_instance" "gabor" { name = "gabor-${count.index + 1}" machine_type = "f1-micro" # get the available zones of the current region and get the number of them #count = "${length(data.google_compute_zones.available.names)}" # pick the zone from the array (we cannot have a count that is larger than the available zones) #zone = "${data.google_compute_zones.available.names[count.index]}" # we can use modulus, but now the number of entries in the list of zones is hard-coded 4 #count = "10" #zone = "${data.google_compute_zones.available.names[count.index % 4]}" #vars.total = "${length(data.google_compute_zones.available.names)}" count = "10" zone = "${data.google_compute_zones.available.names[count.index % ${vars.total} ]}" labels = { owner = "gabor" group = "devops" } }
links
https://www.terraform.io/docs/configuration-0-11/interpolation.html#math
https://www.terraform.io/docs/configuration/resources.html
examples/terraform/google/main.tf
#variable "region" { default = "us-central1" } variable "name" { } variable "zone" { default = "us-central1-a" } provider "google" { project = "project-name-12345" # region = "${var.region}" } resource "google_compute_instance" "gabor" { name = var.name machine_type = "f1-micro" zone = "${var.zone}" boot_disk { initialize_params { image = "ubuntu-1804-lts" } } network_interface { subnetwork = "gce-d-us-central1-indexing" } labels = { activity = "temp" delete_at = "never" group = "devops" owner = "gabor" start_at = "never" stop_at = "never" } connection { type = "ssh" user = "gabor" host = var.name } provisioner "file" { source = "startup.sh" destination = "/home/gabor/startup.sh" } provisioner "remote-exec" { inline = [ "chmod +x /home/gabor/startup.sh" #"apt-get update", #"apt-get install -y htop", ] } metadata = { "startup-script" : "/home/gabor/startup.sh" } #metadata_startup_script = "date >> /opt/test.txt" }
examples/terraform/google/startup.sh
#!/bin/bash -x date >> /home/gabor/startup.log
examples/terraform/google/dev.tfvars
name = "gabor-23" zone = "us-central1-a"
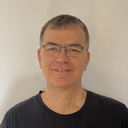
Published on 2019-01-01