Bash shell always succeed with ||: suffix
Trailing ||: on Bash commmands look strange, but they are useful.
If you put that expression at the end of another expression then, even if the first expression fails the whole expression will still succeed, the exit code will be set to 0, and the code will keep running.
See set -e to stop script on failure and set -x to print statements as they are executed
examples/good.sh
#!/bin/bash set -xe ls examples/good.sh echo $?
$ ./examples/good.sh + ls examples/good.sh examples/good.sh + echo 0 0
examples/bad.sh
#!/bin/bash set -xe ls examples/no_such_file.txt echo $?
$ ./examples/bad.sh + ls examples/no_such_file.txt ls: cannot access 'examples/no_such_file.txt': No such file or directory + echo 2 2
examples/bad_without_e.sh
#!/bin/bash set -x ls examples/no_such_file.txt echo $?
$ ./examples/bad_without_e.sh + ls examples/no_such_file.txt ls: cannot access 'examples/no_such_file.txt': No such file or directory + echo 2 2
examples/bad_saved.sh
#!/bin/bash set -xe ls examples/no_such_file.txt ||: echo $?
$ ./examples/bad_saved.sh + ls examples/no_such_file.txt ls: cannot access 'examples/no_such_file.txt': No such file or directory + : + echo 0 0
Explanation
There are two separate pieces of syntax here:
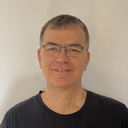
Published on 2021-02-23