Handling user events in JavaScript
In the previous article we saw how can we change the DOM and update the HTML page, but when should we do that? In the previous example the code updating the HTML page ran immediately as it was loaded in the browser.
This time we would like to capture an event generated by the user - a click on a button - and we would like to update the HTML page when that happens.
In JavaScript we can attach various, so called "event listeners" to every DOM element and we can even specify to which event should the specific listener react to.
For this we can use the addEventListener method of a DOM element, that will get two parameters. The first one is the name of the event, such as click or keyup, or change. The second one is a function. (I might need to explain how can you pass a function as a parameter to another function.)
Here is the full expression:
document.getElementById('btn').addEventListener('click', clicked);
This means that when the browser detects a 'click' on the HTML element with the id 'btn', then it will execute the function called 'clicked'.
What does the 'clicked' function do?
It changes the DOM (the HTML) by inserting the text 'Hello World' in the element with the id 'display'. This operation was explained in the article about changing the DOM.
Go ahead try the example by clicking on the Try link.
examples/js/event_listener.html
<button id="btn">Click me</button> <div id="display"></div> <script> function clicked() { document.getElementById('display').innerHTML = 'Hello World'; } document.getElementById('btn').addEventListener('click', clicked); </script>Try!
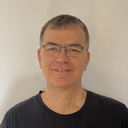
Published on 2015-03-29