Logging in AngularJS applications
We have already seen how to log in plain JavaScript, and even dealt with logging JavaScript objects, but what if we are using AngularJS?
$log in AngularJS
AngularJS comes with a service called $log that is a simple wrapper around the console.log facilities.
In this example we can see that in order to use the $log service we need to use the dependency injection of AngularJS ['$log', function($log) passing the $log object to the function implementing the controller where we would like to use it.
We see both calls to 5 $log.* methods and to the corresponding console.* methods.
examples/angular/logging.js
angular.module('DemoApp', []) .controller('DemoController', ['$log', function($log) { console.debug("Calling console.debug"); console.info("Calling console.info"); console.log("Calling console.log"); console.warn("Calling console.warn"); console.error("Calling console.error"); $log.debug("Some debug"); $log.info("Some info"); $log.log("Some log"); $log.warn("Some warning"); $log.error("Some error"); }]);
The results looks like this in Chrome:
As you can see the results of the corresponding logging functions look the same in AngularJS and pure JavaScript, except that the calls to the $log.* methods don't show accurate line numbers.
That's clearly a drawback and we are going to try to fix this soon, but before that we are going to look at the advantage of the $log facilities over the corresponding console.* functions.
In any case if you'd like to try it yourself, here is the HTML file that will load the above JavaScript file:
examples/angular/logging.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=yes"> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.5/angular.min.js"></script> <script src="logging.js"></script> </head> <body ng-app="DemoApp" ng-controller="DemoController"> <h1>Main Title</h1> </body> </html>Try!
Turn off logging in AngularJS
The only advantage of $log.debug over console.debug I could find is the ability to turn off the debugging printouts.
We can do that through the $logProvider provider in the config directive of AngularJS by adding the following:
.config(['$logProvider', function($logProvider) { $logProvider.debugEnabled(false); // turns off the calls to $log.debug, but not the others }])
This turns off the $log.debug calls, but as you can see in the comment, it does not turn off the other 4 calls of $log. Our code will still print out any of the info, log, warn, and error messages.
See the results:
As far as I know, Angular does not provide a way to turn those off.
The full JavaScript is here:
examples/angular/logging_off.js
angular.module('DemoApp', []) .config(['$logProvider', function($logProvider) { $logProvider.debugEnabled(false); // turns off the calls to $log.debug, but not the others }]) .controller('DemoController', ['$log', function($log) { console.debug("Calling console.debug"); console.info("Calling console.info"); console.log("Calling console.log"); console.warn("Calling console.warn"); console.error("Calling console.error"); $log.debug("Some debug"); $log.info("Some info"); $log.log("Some log"); $log.warn("Some warning"); $log.error("Some error"); }]);
The corresponding HTML file is here:
examples/angular/logging_off.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=yes"> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.5/angular.min.js"></script> <script src="logging_off.js"></script> </head> <body ng-app="DemoApp" ng-controller="DemoController"> <h1>Main Title</h1> </body> </html>Try!
Showing the correct line numbers
There is a way to tell AngularJS to show the correct line numbers. You just need to bind the $log.* functions to the console.* functions. Like this:
$log.debug = console.debug.bind(console);
You will have to do that for each one of the 5 methods separately. In our example we have done it for 2:
examples/angular/logging_lines.js
angular.module('DemoApp', []) .controller('DemoController', ['$log', function($log) { $log.log = console.log.bind(console); $log.debug = console.debug.bind(console); console.debug("Calling console.debug"); console.info("Calling console.info"); console.log("Calling console.log"); console.warn("Calling console.warn"); console.error("Calling console.error"); $log.debug("Some debug"); $log.info("Some info"); $log.log("Some log"); $log.warn("Some warning"); $log.error("Some error"); }]);
The next HTML file can help you try this in your browser.
examples/angular/logging_lines.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, user-scalable=yes"> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.5/angular.min.js"></script> <script src="logging_lines.js"></script> </head> <body ng-app="DemoApp" ng-controller="DemoController"> <h1>Main Title</h1> </body> </html>Try!
Other Solution
While searching for solutions I've bumped into the Blackbox JavaScript Source Files, that can be used to fix the incorrect reporting of line numbers. Unfortunately that's Chrome only.
Conclusion
As it stands I don't see any real advantage for using the logging mechanism provided by AngularJS. I'll stick with calls to console.*.
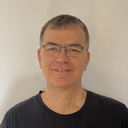
Published on 2016-10-30