Materialize CSS: Side-nav with Vanilla JavaScript on both sides
We already saw how to create a Side-nav with Vanilla JavaScript in Materialize. Now we are goint to create two. A left-side navbar and a right-side navbar.
For more details see the documentation of the sidenav
examples/materializecss/2-side-nav-vanilla-javascript.html
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css"> <meta name="viewport" content="width=device-width, initial-scale=1.0"/> <title>Materialize: Sidenav with vanilla JavaScript</title> </head> <body> <div class="container"> <!-- start the definition of the sidenav --> <ul id="main-slide-out" class="sidenav"> <li><a href="#!">Link</a></li> <li><div class="divider"></div></li> <li><a class="subheader">Subheader</a></li> <li><a href="#!">Other link</a></li> <li>Outdented Title <ul> <li><a href="#!">Alfa</a></li> <li><a href="#!">Beta</a></li> </ul> </li> <li><div class="divider"></div></li> <li><a href="">Title</a> <ul> <li><a href="#!">Alfa</a></li> <li><a href="#!">Beta</a></li> </ul> </li> </ul> <ul id="secondary-slide-out" class="sidenav"> <li><a class="subheader">Secondary</a></li> <li><div class="divider"></div></li> <li><a href="#!">Yet another link</a></li> </ul> <!-- end the definition of the sidenav --> <div class="row"> <!-- the button to open the sidenav --> <div class="col s1"><a href="#" data-target="main-slide-out" class="sidenav-trigger"><i class="material-icons">show main menu</i></a></div> <div class="col s1"><a href="#" data-target="secondary-slide-out" class="sidenav-trigger"><i class="material-icons">show secondary menu</i></a></div> <div class="col s11"> </div> <div class="col s12"> "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum." </div> </div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script> </body> </html> <script> document.addEventListener('DOMContentLoaded', function() { const elem1 = document.getElementById('main-slide-out'); const options1 = { 'edge': 'left' }; const instance1 = M.Sidenav.init(elem1, options1); // instance1.open() const elem2 = document.getElementById('secondary-slide-out'); const options2 = { 'edge': 'right' }; const instance2 = M.Sidenav.init(elem2, options2); // instance2.open(); }); </script>Try!
In order to open the left-side navigation bar the user needs to click on the "show main menu" link. In order to open the right-side navigation bar the user needs to click on the "show secondary menu" link.
The bar automatically closes when the user clicks on the content of the page outside the navbar.
The main by sidenav is aligned to the left side of the screen. The secondary is aligned to the right-side of the screen.
At the bottom of the file you can see the JavaScript code. In this example we searched for the navbars one-by-one using their ID and then initialized them separately using (slightly) different options.
I also included a commented out line of code that would open the navbars when the page is loaded.
You could also use the open method if you wanted to open the secondary navbar when the user selected something on the primary navbar.
In this example I also tried to add a second level of hierachy to the links in the sidenav. It was not really successful. In the first case I had a title that was not a link itself and it got outdented. In the second case everything was just in the same column so the hiearachy is not visible to the users.
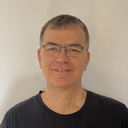
Published on 2022-08-17