Materialize CSS: Side-nav with Vanilla JavaScript
Materialize has several features that require the use of JavaScript. One of the is the side navigation bar.
For more details see the documentation of the sidenav
examples/materializecss/side-nav-vanilla-javascript.html
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css"> <meta name="viewport" content="width=device-width, initial-scale=1.0"/> <title>Materialize: Sidenav with vanilla JavaScript</title> </head> <body> <div class="container"> <!-- start the definition of the sidenav --> <ul id="slide-out" class="sidenav"> <li><a href="#!">Link</a></li> <li><div class="divider"></div></li> <li><a class="subheader">Subheader</a></li> <li><a href="#!">Other link</a></li> </ul> <!-- end the definition of the sidenav --> <div class="row"> <!-- the button to open the sidenav --> <div class="col s1"><a href="#" data-target="slide-out" class="sidenav-trigger"><i class="material-icons">show menu</i></a></div> <div class="col s11"> </div> <div class="col s12"> "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum." </div> </div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script> </body> </html> <script> document.addEventListener('DOMContentLoaded', function() { const elems = document.querySelectorAll('.sidenav'); const options = { 'edge': 'right' }; var instances = M.Sidenav.init(elems, options); // Enable this to make the side-nav open automatically when page is loaded. // instances[0].open(); }); </script>Try!
In order to open the navigation bar the user needs to click on the "show menu" link. The bar automatically closes when the user clicks on the content of the page outside the navbar.
By default the navbar is aligned to the left side of the screen. In our example we used the "edge" field of the "options" to make it appear on the right side.
At the bottom of the file you can see the JavaScript code. In this example we searched for all the navbars (even though we only have one in our example) and then initialized all of them by a single call using the same options. If you have more than one navbar you could locate each one of them by their id and initialize each one of them with different options.
I also included a commented out line of code that would open the navbar when the page is loaded.
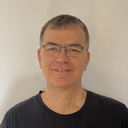
Published on 2022-08-17