ARGV - the command line arguments of a Ruby program
When you run a script written in Ruby you can put all kinds of values on the command line after the name of the script:
For example:
ruby code.rb abc.txt def.txt qqrq.txt
or like this:
ruby code.rb Hello --machine big -d -tl
The question though, how can the Ruby program know what was given on the command line?
Ruby maintains an array called ARGV with the values passed on the command line. We can access the elements of this array, just as any other array:
ARGV[0] is going to be the first value after the name of the script.
We can iterate over the elements either directly with a for loop:
examples/ruby/command_line_argv.rb
for arg in ARGV puts arg end
or iterating over the range of indexes, and accessing the elements using that index.
examples/ruby/command_line_argv_with_index.rb
for i in 0 ... ARGV.length puts "#{i} #{ARGV[i]}" end
$ ruby command_line_argv_with_index.rb foo bar --machine big 0 foo 1 bar 2 --machine 3 big
Verifying the number of arguments
For a simple input validation we can check the length of the ARGV array. Report if we have not received enough arguments and exit the program early.
examples/ruby/command_line_argv_check_length.rb
if ARGV.length < 2 puts "Too few arguments" exit end puts "Working on #{ARGV}";
Running this script we get:
$ ruby command_line_argv_check_length.rb one Too few arguments $ ruby command_line_argv_check_length.rb one two Working on ["one", "two"]
Values received on the command line are strings
In this snippet of code we first check if we got exactly 2 parameters and we do, we add them together:
examples/ruby/command_line_argv_add.rb
if ARGV.length != 2 puts "We need exactly two arguments" exit end puts ARGV[0] + ARGV[1]
ruby command_line_argv_add.rb 23 19 2319
The result might not be surprising to you if you know that the values the user passes on the command line are received as strings. Eeven if they are actually numbers. If we would like to use them as number we have to convert them using to_i:
examples/ruby/command_line_argv_add_numbers.rb
if ARGV.length != 2 puts "We need exactly two arguments" exit end puts ARGV[0].to_i + ARGV[1].to_i
$ ruby command_line_argv_add_numbers.rb 23 19 42
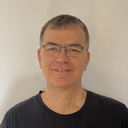
Published on 2015-10-06