Reading CSV file in Ruby
Ruby comes with a standard library called CSV to make it easy to read files with Comman Separated values
CSV file
In this CSV file the 3rd fields in every "row" is a number. We would like to sum these numbers.
examples/data/distance.csv
Budapest,Bukarest,1200,km Tel Aviv,Beirut,500,km London,Dublin,300,km New York,"Moscow, East",6000,km Local,"Remote Location",10,km
If it was a simpler file We could read it line-by-line and use split to cut it into parts, but in this file there is a field that has a comman in it. Plain split would not be able to handle the field enclosed in quote marks " containing a comma.
This file also has a field with an embedded newline. So the physical rows of the file, marked by \n newlines are not the same as the logical lines that and good CSV parser would understand.
examples/ruby/add_third_column.rb
require "csv" filename = File.dirname(File.dirname(File.expand_path(__FILE__))) + '/data/distance.csv' sum = 0 CSV.foreach(filename) do |row| sum += row[2].to_i end puts sum
In this example first we load the CVS module then we use the CVS.foreach(filename) construct to iterate over the file loical row by logical row. On each iteration the variable row is going to be an array. The third element can be accessed using index 2. We have to convert the value to a number using to_i and then we can add it to the variable sum
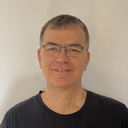
Published on 2015-10-09