Basic data structures in Ruby (Scalar, Array, Hash)
In Ruby there are 3 basic data structures.
Scalars can hold a single value: a number or string.
Arrays is an ordered list of scalars.
Hashes are key-value pairs where the keys are uniques strings and the values are scalars
The class method can tell us what kind of value a variable contains:
examples/ruby/data_structures.rb
x = 43 puts x.class # Fixnum q = 3.14 puts q.class # Float z = "abc" puts z.class # String colors = [ 'Blue', 'Green', 'Yellow' ] puts colors.class # Array person = { 'fname' => 'Foo', 'lname' => 'Bar' } puts person.class # Hash
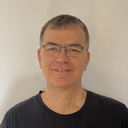
Published on 2015-10-12