split in Ruby
String objects in Ruby have a method called split. It is similar to the split function of Perl. It can cut up a string into pieces along a pre-defined string or regex returning an array of smaller strings.
In the first example you can see how to split a string every place where there is a comma ,:
examples/ruby/split_comma.rb
require 'pp' words_str = 'Foo,Bar,Baz' words_arr = words_str.split(',') pp words_arr # ["Foo", "Bar", "Baz"]
In the second example we use a Regex to match the places where we would like to cut up the string. It makes the splitting much more flexible:
examples/ruby/split_regex.rb
require 'pp' words_str = 'One - Two- Three' words_arr = words_str.split(/\s*-\s*/) # ["One", "Two", "Three"] pp words_arr
Split with limit
We can pass a second parameter to split that will limit the number of reurned valus. If we pass 3, then split will make two cuts and return the results:
examples/ruby/split_comma_limit.rb
require 'pp' words_str = 'Foo,Bar,Baz,Moo,Zorg' words_arr = words_str.split(',', 3) pp words_arr # ["Foo", "Bar", "Baz,Moo,Zorg"]
Split by empty string
As a slightly special case, if we use an empty string (or empty regex) to split with, then we will get back an array of the individual characters:
examples/ruby/split_by_empty_string.rb
require 'pp' words_str = 'Foo,Bar,Baz' words_arr = words_str.split('') pp words_arr # ["F", "o", "o", ",", "B", "a", "r", ",", "B", "a", "z"]
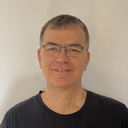
Published on 2015-10-31