Functions in Linux shell (bash)
Define and call a function in Bash
examples/shell/hello_world_function.sh
function hello() { echo "Hello World" } echo before hello echo after
Return value from shell function
examples/shell/return_from_function.sh
function hello() { echo "hello world" } result=$(hello) echo before echo $result echo after
Parameter passing to functions in shell
examples/shell/parameter_passing.sh
function foo() { echo "param: '$1' param: '$2'" } echo before foo "one" "and two" echo after
Early return from shell functions
examples/shell/early_return.sh
function foo() { param=$1 if [ "$param" == "ok" ] then echo "short" return fi echo "long" } foo foo ok foo
See also Returning Values from Bash Functions
Empty shell functions
examples/shell/empty.sh
function hello() { }
This does not work, but gives this error:
./empty.sh: line 3: syntax error near unexpected token `}' ./empty.sh: line 3: `}'
examples/shell/empty_with_colon.sh
function hello() { : }
A Bash function can be called only after declaration
# qqrq # ./func.sh: line 2: qqrq: command not found function qqrq() { echo "hello" } qqrq
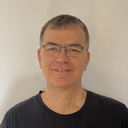
Published on 2019-01-17