How to commafy a number? (How to print number with commas as thousands separators using Python?)
examples/python/commafy.py
from __future__ import print_function small = 42 big = 1234567 print(small) # 42 print(big) # 1234567 print("{:,}".format(small)) # 42 print("{:,}".format(big)) # 1,234,567
We have imported the print_function from the __future__ so this code will work on both Python 2 and Python 3.
The key here is the {:,} placeholder.
Commafy in Jinja and Flask
We can create new filters for Jinja and add them to the environment:
examples/python/jinja-commafy/generate_from_filesystem.py
from jinja2 import Environment, FileSystemLoader import os root = os.path.dirname(os.path.abspath(__file__)) env = Environment( loader = FileSystemLoader(root) ) env.filters['commafy'] = lambda v: "{:,}".format(v) template = env.get_template('page.html') print(template.render( distance = 12345678, ))
In the template we can filter values using this new filter:
examples/python/jinja-commafy/page.html
{{ distance }} {{ distance | commafy }}
The output will look like this:
12345678 12,345,678
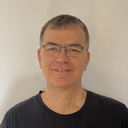
Published on 2016-08-16