Jenkins Pipeline parameters
Jenkins pipelines can declare what kind of parameters it accepts and what are the defaults to those parameters.
Scheduled jobs will use the default values. Users running the job manually can set the parameters.
examples/jenkins/parameters.Jenkinsfile
pipeline { agent { label 'master' } parameters { string(name: 'hostname', defaultValue: 'gabor-dev', description: 'Hostname or IP address') booleanParam(name: 'yesno', defaultValue: false, description: 'Checkbox') choice(name: 'planet', choices: ['Mercury', 'Venus', 'Earth', 'Mars'], description: 'Pick a planet') choice(name: 'space', choices: ['', 'Mercury', 'Venus', 'Earth', 'Mars'], description: 'Pick a planet. Defaults to empty string') text(name: 'story', defaultValue: 'One\nTwo\nThree\n', description: '') password(name: 'secret', defaultValue: '', description: 'Type some secret') } stages { stage('display') { steps { echo params.hostname echo params.yesno ? "yes" : "no" echo params.planet echo params.space echo params.story //echo params.secret echo "--------" echo "${params.hostname}" echo "${params.yesno}" echo "${params.planet}" echo "${params.space}" echo "${params.story}" echo "${params.secret}" script { sh "echo ${params.secret}" } } } } }
Trying to echo params.secret caused an exception
java.lang.ClassCastException: org.jenkinsci.plugins.workflow.steps.EchoStep.message expects class java.lang.String but received class hudson.util.Secret
Similarly echo params.yesno
raised this exception:
java.lang.ClassCastException: org.jenkinsci.plugins.workflow.steps.EchoStep.message expects class java.lang.String but received class java.lang.Boolean
Putting them in string solved the problem for both of them.
I also included a small script that uses the Linux shell to echo the value.
Primarily shoing that even though the content of a password field will not be visible on the UI of Jenkins, the console log might contain it if we are not careful. Better not to print it.
See documentation
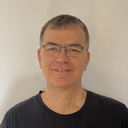
Published on 2020-12-07