SVG Rectangle
This is part of the SVG Tutorial and exercises drawing a rectangle in SVG.
The solution to the rectangle exercise as listed on SVG page:
examples/js/svg_rectangle.js
function draw_rectangle() { var draw = SVG('blue_rectangle'); draw.size(200, 100); var rect = draw.rect(200, 100); rect.attr({ fill: '#00f' }); } draw_rectangle();
Drawing Rectangles
In the next section we go over various attributes you can set on a rectangle. Many of these are relevant to every other shape as well.
Default rectangle
In this example we just create an SVG image and then create a rectangle of the same size.
examples/js/svg_rectangle_1.js
function rect_1() { var img = SVG('rect_1'); img.size(200, 100); // width, height var rect = img.rect(200, 100); // width, height } rect_1();
White rectangle
examples/js/svg_rectangle_2.jsfunction rect_2() { var img = SVG('rect_2'); img.size(200, 100); var rect = img.rect(200, 100); rect.fill({ color: '#FFF' }); } rect_2();
Of course, on a white background it is hard to see the white rectangle...
Nice rectangle
Let's give it some other color:
examples/js/svg_rectangle_3.js
function rect_3() { var img = SVG('rect_3'); img.size(200, 100); var rect = img.rect(200, 100); rect.fill({ color: '#F06' }); } rect_3();
White rectangle with border
Alternatively, we can have the background in white, but we can add a border. We use stroke for this:
examples/js/svg_rectangle_4.js
function rect_4() { var img = SVG('rect_4'); img.size(200, 100); var rect = img.rect(200, 100); rect.fill({ color: '#FFF' }).stroke({ width: 1 }); } rect_4();
Smaller rectangle
Up until now the rectangle we drew was the same size as the image itself. Now we are going to see how a smaller rectangle can be placed on that image. In order to make it clearer we actually use two rectangles. The first one created by img.rect(200, 100).fill({ color: '#FFF' }).stroke({ width: 1 }); provides the background with a 1 pixel wide border. That will make it easier to see what happens to the other rectangle we draw.
The second rectangle is smaller (only 100 pixel wide and 50 pixel high) and it has that nice color we had earlier.
As you can see the smaller rectangle is attached to the top-left corner of the image. That's because the point at (x,y) coordinates (0,0) in SVG is the top left corner.
examples/js/svg_rectangle_5.js
function rect_5() { var img = SVG('rect_5'); img.size(200, 100); img.rect(200, 100).fill({ color: '#FFF' }).stroke({ width: 1 }); var rect = img.rect(100, 50); rect.fill({ color: '#F06' }); } rect_5();
Move the SVG element on the x and y axes
Let's put the rectangle in some other place. There are several ways to indicate the location of an SVG element. The x() and y() methods allow us to set the coordinates of (the top-left corner of) a rectangle to absolute values on the x,y axes.
In this example we have moved the rectangle 30 pixels to the right on the x axis, and 5 pixels down on the y axis.
We could call x(30) several times, on the same object, only the last call would matter.
If we call x() or y() with a parameter as above, they both return the current object, which means we can stack the calls one on the other as we do in the examples.
On the other hand, calling x() or y() without any parameter will return the current location of the object on the x,y axes.
examples/js/svg_rectangle_6.js
function rect_6() { var img = SVG('rect_6'); img.size(200, 100); img.rect(200, 100).fill({ color: '#FFF' }).stroke({ width: 1 }); var rect = img.rect(100, 50); rect.fill({ color: '#F06' }).x(30).y(5); } rect_6();
Move the element relative to its own position
The dx() and dy() methods allow us to move the object relative to its current position. When the object is created it does not matter if we use x() or dx() (at least on rectangles) as the default location of the rectangle is 0,0, but later we'll see how we can change the coordinates of an existing object. Then it will be important that we can either set the coordinates in absolute terms using x() and y(), or in relative terms using dx() and dy().
In the example we can see that we call dy() multiple times stacked one on the other and the movements add up.
examples/js/svg_rectangle_7.js
function rect_7() { var img = SVG('rect_7'); img.size(200, 100); img.rect(200, 100).fill({ color: '#FFF' }).stroke({ width: 1 }); var rect = img.rect(100, 50); rect.fill({ color: '#F06' }).dx(30).dy(5).dy(5).dy(5); } rect_7();
Positioning by the center of the object
Sometimes it is easier to position an element by its center. Using cx() and cy is quite similar to using x() and y(), except that the coordinates we give are that of the center of the rectangle. For example if we set the center to 0, 0 then we'll only see one quarter of the rectangle:
examples/js/svg_rectangle_8.js
function rect_8() { var img = SVG('rect_8'); img.size(200, 100); img.rect(200, 100).fill({ color: '#FFF' }).stroke({ width: 1 }); var rect = img.rect(100, 50); rect.fill({ color: '#F06' }).cx(0).cy(0); } rect_8();
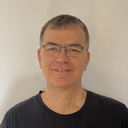
Published on 2015-02-14