SVG - Scalable Vector Graphics with JavaScript
On a computer, every image is made out of pixels in various colors. If the image is very smooth then we have a lot of pixels. Still just pixels.
If we want to save a picture to a file basically we can have two options. Either we save the pixels which is called raster image, or we store instructions how to draw lines in order to generate the pixels. This is called vector image.
The advantage of vector graphics is that we can enlarge the image without loss of quality.
Scalable Vector Graphics (SVG) is an XML file containing instructions to draw an image. SVG.JS is a JavaScript library that makes it easy to create such XML file and even without saving them to disk, to use them to draw images on a web site.
Just as we saw a examples for SVG images drawn with Perl, let's see a few simple examples with the JavaScript library.
Download the implementation of SVG.JS using wget https://raw.github.com/wout/svg.js/master/dist/svg.min.js or some other way and let's start drawing.
Square
Create the following two files:
An HTML file that loads the svg.min.js we have just downloaded. This can go anywhere in the HTML file.
Then we add an HTML element with an id. (square_1 in our case). This is where the drawing will be placed.
Finally we load a JavaScript file where we put the instructions. Unless we employ some kind of delayed execution, this JavaScript file has to come after the other two.
examples/js/svg_square.html
<script src="svg.min.js"></script> <div id="square_1"></div> <script src="svg_square.js"></script>Try!
The other file is the JavaScript file with the instruction. The SVG() function gets the id of the HTML element where we want to put our drawing. This returns an object. On this object we can call various methods that will impact the drawing.
examples/js/svg_square.js
function draw_square() { var draw = SVG('square_1'); draw.size(120, 120); var square = draw.rect(100, 100); square.attr({ fill: '#f06' }); } draw_square()
The result will look like this:
Circle
In order to draw a circle it is enough to provide its diameter:
examples/js/svg_circle.js
function draw_circle() { var draw = SVG('circle_1'); draw.size(120, 120); draw.circle(100).attr({ fill: '#32AD4F' }); } draw_circle();
And have the appropriate HTML file to load the JavaScript:
examples/js/svg_circle.html
<div id="circle_1"></div> <script src="svg.min.js"></script> <script src="svg_circle.js"></script>Try!
Polygon
examples/js/svg_polygon.js
var draw_polygon_1 = SVG('polygon_1'); draw_polygon_1.size(120, 120); var polygon = draw_polygon_1.polygon('0,0 100,50 50,100'); polygon.fill('#2ABFB5').stroke({ width: 3 })
And have the appropriate HTML file to load the JavaScript:
examples/js/svg_polygon.html
<div id="polygon_1"></div> <script src="svg.min.js"></script> <script src="svg_polygon.js"></script>Try!
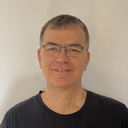
Published on 2015-02-13