Creating PDF files using Python and reportlab
Install the reportlab module using:
pip install reportlab
Use the canvas.Canvas to create the skeleton.
Use drawString to add a string.
I admit, I don't know why do I need to call showPage as it seemed to work without that too. I'll updated this post when I find it out.
save will save the pdf file.
examples/python/pdf_hello_world.py
from reportlab.pdfgen import canvas pdf_file = 'hello_world.pdf' can = canvas.Canvas(pdf_file) can.drawString(20, 400, "Hello World!") can.showPage() can.save()
Coordinates, sizes
By default the coordinates of a string are provided in pixels, starting from the lower left corner which is 0, 0.
You can use the reportlab.lib.units module that provides objects such as 'cm', 'inch', 'mm', 'pica', 'toLength' to use more human-friendly units.
examples/python/reportlab_lib_units.py
from reportlab.lib.units import cm, inch, mm, pica, toLength print(cm) # 28.346456692913385 print(inch) # 72.0 print(mm) # 2.834645669291339 print(pica) # 12.0
You'd use them like this:
examples/python/pdf_hello_world_cm.py
from reportlab.pdfgen import canvas from reportlab.lib.units import cm pdf_file = 'hello_world_cm.pdf' can = canvas.Canvas(pdf_file) can.drawString(2*cm, 20*cm, "Hello World!") can.showPage() can.save()
Fonts (types and sizes)
The getAvailableFonts method will return the list of available fonts.
You can use the setFont method to set the font-type and size. From that point till the next call of setFont, this will be used.
examples/python/pdf_hello_world_fonts.py
from reportlab.pdfgen import canvas pdf_file = 'hello_world_fonts.pdf' can = canvas.Canvas(pdf_file) print(can.getAvailableFonts()) # 'Courier', 'Courier-Bold', 'Courier-BoldOblique', 'Courier-Oblique', # 'Helvetica', 'Helvetica-Bold', 'Helvetica-BoldOblique', 'Helvetica-Oblique', # 'Symbol', # 'Times-Bold', 'Times-BoldItalic', 'Times-Italic', 'Times-Roman', # 'ZapfDingbats' can.setFont("Helvetica", 24) can.drawString(20, 400, "Hello") can.drawString(40, 360, "World") can.setFont("Courier", 16) can.drawString(60, 300, "How are you?") can.showPage() can.save()
Default page size
examples/python/pdf_default_pagesize.py
from reportlab.pdfgen import canvas from reportlab.rl_config import defaultPageSize print(defaultPageSize) # (595.2755905511812, 841.8897637795277) WIDTH, HEIGHT = defaultPageSize pdf_file = 'pagesize.pdf' can = canvas.Canvas(pdf_file) can.drawString(WIDTH/4, HEIGHT-20, "WIDTH:{} HEIGHT:{}".format(WIDTH, HEIGHT)) can.showPage() can.save()
For further details and explanation see the reportlab userguide.
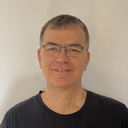
Published on 2019-10-19