Time left in process (progress bar) in Python
In a project some Python code was used to monitor the progress of another process. Besides reporting the progress basd on the number of units processed so far, I wanted to be able to show how much time is left in the process.
We knew how many items (units) are there to process, every once in a while we could check how many have been alread processed (by looking up in a database).
The same can be used even if the processing is done in the same program where we have the monitoring.We just need to calculate how much time - on average - took to process one unit (dividing the elapsed time by the number of units done) and then multiplying it by the number of units remaining.
It won't be exact, but can give you a better feeling to see how much more you have to wait.
examples/python/time_left.py
import time import datetime class Timer(object): def __init__(self, total): self.start = datetime.datetime.now() self.total = total def remains(self, done): now = datetime.datetime.now() #print(now-start) # elapsed time left = (self.total - done) * (now - self.start) / done sec = int(left.total_seconds()) if sec < 60: return "{} seconds".format(sec) else: return "{} minutes".format(int(sec / 60)) # There are 12987 units in this task t = Timer(12987) # do some work time.sleep(1) # after some time passed, or after some units were processed # eg. 37 units, calculate, and print the remaining time: print(t.remains(37)) # let the process continue and once in a while print the remining time.
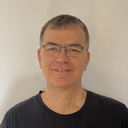
Published on 2018-08-22