Static code analysis for Python code - PEP8, FLAKE8, pytest
Install
pip install flake8 pip install flake8-builtins pip install pytest-flake8
Run
pytest --flake8 .
If you'd like to run the static analysis on the directory where you have the tests files, and if you want the test-run to only include the flake8 tests then you need a way to exclude all the other tests. For this I can recommend you use a fake marker. That is, using -m qqrq you tell pytest that only run the test-functions that have a marker "qqrq". Hopefully none of your tests will use this marker and thus none of your tests will run.
Only the flake8 static code analysis.
pytest --flake8 -m qqrq .
Configure by including the following in the setup.cfg file in the root of your project.
examples/setup-with-flake8.cfg
[tool:pytest] flake8-ignore = *.py E123 E124 E126 E127 E128 E201 E202 E203 E211 E221 E222 E241 E251 E262 E265 E266 E305 E402 E501 W191 W291 W293 W391 F401 F811 F841 W605 some_dir/*.py ALL addopts = -p no:cacheprovider
PEP8
Flake already includes PEP8, so the following is only needed if for some reason you cannot use flake8:
pip install pytest-pep8
examples/setup-with-pep8.cfg
[tool:pytest] pep8maxlinelength = 2300 pep8ignore = *.py E123 E124 E126 E127 E128 E129 E201 E202 E203 E221 E241 E251 E262 E265 E266 E402 some_dir/*.py ALL addopts = -p no:cacheprovider
Cyclomtic complexity
Use-case for flake8
data = 42 other = "Data: {}".format(data)
Converted to:
data = 42 other = "Data: {data}"
forgetting the "f" of the f-string. Now we have a variable called data that is not in use. Flake8 will report: F841 local variable 'data' is assigned to but never used
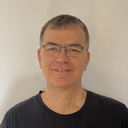
Published on 2019-09-21