Simple in-memory counter with AngularJS
In the big counter example we see a number of implementations of counters. Here is one using AngularJS.
Simple button to increment counter
examples/angular/in_memory_counter.html
<script src="angular.min.js"></script> <div ng-app> <button ng-init="counter = 0" ng-click="counter = counter + 1">Increment</button> {{counter}} </div>Try!
In this example we have an HTML button that has two Angular attributes. ng-init will be executed once when the page loads. It gives the initial value to the attribute counter.
The content of ng-click will be executed every time we click on the button. That will increment the counter by 1. (counter++ does not work here)
When we load the page we can see the button "Increment" and the number 0. Then as we click on the button the number gets incremented.
Increment and decrement buttons
In the next example we have added another button to decrement the counter by 1. In addition, in order to make the step clearly separated, we have moved the ng-init attribute to a separate div element which is not going to be displayed at all.
examples/angular/in_memory_counter_with_decrement.html
<script src="angular.min.js"></script> <div ng-app> <div ng-init="counter = 0"></div> <button ng-click="counter = counter + 1">Increment</button> <button ng-click="counter = counter - 1">Decrement</button> {{counter}} </div>Try!
In-memory counter with controller
In preparation from some more substantial actions behind the counter, in the third example we have move the code decrementing the counter to a controller. (The button incrementing the counter was left as it was before.)
This time we've created an Angular module and controller, in which we set the default value of $scope.counter to 0 and we have also defined a method called decrement. As this is already plain JavaScript, here we can already use the autoincrement and autodecrement expression like this one: counter--.
In the HTML we set ng-click="decrement()" which means the decrement method will be called every time a button is pressed.
examples/angular/in_memory_counter_with_controller.html
<script src="angular.min.js"></script> <script> angular.module("CounterApp", []) .controller("CounterController", function($scope) { $scope.counter = 0; $scope.decrement = function() { $scope.counter--; }; }) </script> <div ng-app="CounterApp"> <div ng-controller="CounterController"> <button ng-click="counter = counter + 1">Increment</button> <button ng-click="decrement()">Decrement</button> {{counter}} </div> </div>Try!
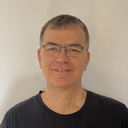
Published on 2015-08-05