Groovy: Date, Time, Timezone
examples/groovy/date.gvy
d = new Date() println(d) println(d.getTime()) // epoch d += 1 // increment days by 1 println(d) println(d.next()) // next day (incement by 1) println(d) println(d.seconds) println(d.minutes) println(d.hours) println(d.day) println(d.month) println(d.year) e = d // create a copy e += 3 // increment by 3 days println(e) println(d) z = e-d println(z) // 3
examples/groovy/date.out
Mon Jan 06 20:55:37 IST 2020 1578336937753 Tue Jan 07 20:55:37 IST 2020 Wed Jan 08 20:55:37 IST 2020 Tue Jan 07 20:55:37 IST 2020 37 55 20 2 0 120 Fri Jan 10 20:55:37 IST 2020 Tue Jan 07 20:55:37 IST 2020 3
Adjust Timezone in Groovy
While normally Jeruslame is at UTC+2, during the daylight saving time, it is actually UTC+3. This is reflected in the results.
examples/groovy/time_zone.gvy
import java.util.TimeZone tz = TimeZone.getTimeZone("Asia/Jerusalem") def ts = new Date() println(ts) // 12:43:14 UTC 2018 println(ts.format("HH:mm")) // 12:43 println(ts.format("HH:mm", timezone=tz)) // 15:43
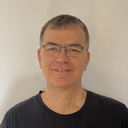
Published on 2018-09-07
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post