Groovy Exception handling (try, catch, Exception)
examples/groovy/divide.groovy
def div(a, b) { return a/b } if (args.size() < 2) { println("You need to pass two numbers") System.exit(1) } def res = div(args[0] as Integer, args[1] as Integer) println(res)
It works well if the division work well, but:
$ groovy divide.groovy 3 0 Caught: java.lang.ArithmeticException: Division by zero java.lang.ArithmeticException: Division by zero at divide.div(divide.groovy:2) at divide.run(divide.groovy:13)
We can use try and catch to catch the exception:
examples/groovy/catch_exception.groovy
def div(a, b) { return a/b } if (args.size() < 2) { println("You need to pass two numbers") System.exit(1) } try { def res = div(args[0] as Integer, args[1] as Integer) println(res) } catch(Exception e) { println("Exception: ${e}") }
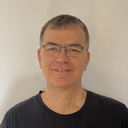
Published on 2019-04-21
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post