Groovy pop push
In Groovy you can use an array as if it was a stack pushing elements to the end and poping elements from the end.
examples/groovy/push_pop.groovy
names = [] names.push('Mary') names.push('Joe') names.push('Fanny') names.push('George') names.push('Liz') names.push('Peter') println(names) first = names.pop() println(first) println(names)
[Mary, Joe, Fanny, George, Liz, Peter] Peter [Mary, Joe, Fanny, George, Liz]
Pop from empty array raises exception
examples/groovy/pop_empty.groovy
names = [] names.pop()
Caught: java.util.NoSuchElementException: Cannot pop() an empty List java.util.NoSuchElementException: Cannot pop() an empty List at pop_empty.run(pop_empty.groovy:2)
Check emptyness before calling pop
To avoid the exception we can check the size of the array before calling pop.
examples/groovy/pop_check_empty.groovy
names = [] if (names.size()) { println(names.pop()) }
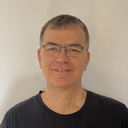
Published on 2019-04-20