Groovy throw (raise) exception
Being able to catch exceptions is important, but so is the ability to raise exceptions (or throw exceptions) as it is called in Groovy.
If you pass a negative number to the Math.sqrt method, it will return a value called NaN Not A Number.
examples/groovy/sqrt.groovy
println( Math.sqrt( 4 )) // 2.0 println( Math.sqrt( -1 )) // NaN println('still alive') // still alive
What if you'd like to write your own sqrt function that will throw an exception if a negative value is passed to it?
Here is a solution:
examples/groovy/my_sqrt.groovy
def sqrt(int n) { if (n < 0) { throw new Exception("The number ${n} was negative") } return Math.sqrt(n) } println( sqrt( 4 )) // 2.0 println( sqrt( -1 )) // exception println('still alive') // is not executed...
2.0 Caught: java.lang.Exception: The number -1 was negative java.lang.Exception: The number -1 was negative at my_sqrt.sqrt(my_sqrt.groovy:4) at my_sqrt$sqrt.callCurrent(Unknown Source) at my_sqrt.run(my_sqrt.groovy:11)
The code that would print "still alive" is not executed.
Catch my exception
examples/groovy/catch_my_sqrt.groovy
def sqrt(int n) { if (n < 0) { throw new Exception("The number ${n} was negative") } return Math.sqrt(n) } println( sqrt( 4 )) // 2.0 try { println( sqrt( -1 )) } catch(Exception e) { println("Exception: ${e}") } // Exception: java.lang.Exception: The number -1 was negative println('still alive') // still alive
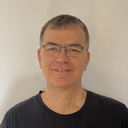
Published on 2019-04-21