Groovy: JSON - reading and writing
Parse JSON string
We can use the JsonSlurper class to parse JSON strings.
If we have JSON string in a Groovy variable we can parse it to become a Groovy map:
examples/groovy/parsing_json.groovy
import groovy.json.JsonSlurper def json_str = '''{ "name": "Foo Bar", "year": 2018, "timestamp": "2018-03-08T00:00:00", "tags": [ "person", "employee" ], "grade": 3.14 }''' def jsonSlurper = new JsonSlurper() cfg = jsonSlurper.parseText(json_str) println(cfg) // [name:Foo Bar, year:2018, timestamp:2018-03-08T00:00:00, tags:[person, employee], grade:3.14] println(cfg['name']) // Foo Bar println(cfg.name) // Foo Bar
Creating JSON string
JsonOutput has several methods. toJson returns a JSON string in one line. prettyPrint returns a nicely formatted JSON string. The latter takes up more space, but it is also human-readable.
examples/groovy/create_json.groovy
import groovy.json.JsonOutput def data = [ name: "Foo Bar", year: "2018", timestamp: "2018-03-08T00:00:00", tags: [ "person", "employee"], grade: 3.14 ] def json_str = JsonOutput.toJson(data) println(json_str) def json_beauty = JsonOutput.prettyPrint(json_str) println(json_beauty)
In the output you can see both the result of toString and the result of prettyPrint.
examples/groovy/create_json.txt
{"name":"Foo Bar","year":"2018","timestamp":"2018-03-08T00:00:00","tags":["person","employee"],"grade":3.14} { "name": "Foo Bar", "year": "2018", "timestamp": "2018-03-08T00:00:00", "tags": [ "person", "employee" ], "grade": 3.14 }
Read JSON from file
examples/groovy/read_json.groovy
import groovy.json.JsonSlurper if (args.size() < 1) { println("Missing filename") System.exit(1) } filename = args[0] def jsonSlurper = new JsonSlurper() data = jsonSlurper.parse(new File(filename)) println(data)
The parse method accepts a File object, reads in the content of the file and then parses it.
Write JSON file
In order to write a JSON file, you need to create the JSON string (either as a plain string or as a beautified string) and then use the File class to save it.
examples/groovy/write_json.groovy
import groovy.json.JsonOutput if (args.size() < 1) { println("Missing filename") System.exit(1) } filename = args[0] def data = [ name: "Foo Bar", year: "2018", timestamp: "2018-03-08T00:00:00", tags: [ "person", "employee"], grade: 3.14 ] def json_str = JsonOutput.toJson(data) def json_beauty = JsonOutput.prettyPrint(json_str) File file = new File(filename) file.write(json_beauty)
More JSON
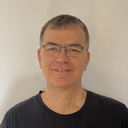
Published on 2018-08-02