Groovy map (dictionary, hash, associative array)
Groovy map access elements
g = [:] // create map println(g) g['name'] = [] // create array println(g) g['name'].add("foo") g['name'].add("bar") println(g)
examples/groovy/elements_of_map.groovy
def colors = [red: '#FF0000', green: '#00FF00', blue: '#0000FF'] println colors println colors.containsKey('red') println colors.containsKey('black') for (p in colors) { println p.key println p.value }
Get all the keys ina Groovy map
examples/groovy/keys_of_map.groovy
def colors = [red: '#FF0000', green: '#00FF00', blue: '#0000FF'] for (key in colors.keySet()) { println(key) println(colors[key]) }
red #FF0000 green #00FF00 blue #0000FF
Sorted keys of a Groovy map
examples/groovy/sorted_keys_of_map.groovy
def colors = [red: '#FF0000', green: '#00FF00', blue: '#0000FF'] for (key in colors.keySet().sort()) { println(key) println(colors[key]) }
Values of a Groovy map
examples/groovy/sorted_keys_of_map.groovy
def colors = [red: '#FF0000', green: '#00FF00', blue: '#0000FF'] for (key in colors.keySet().sort()) { println(key) println(colors[key]) }
Return map from a function
examples/groovy/return_map.gvy
def f() { return ["a": 2, "b": 3] } r = f() println r['a'] println r['b']
See also maps
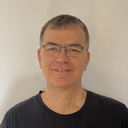
Published on 2018-07-31
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post