Count digits in Groovy
In the count digit exercise we need to take a file like this:
examples/data/count_digits.txt
23 34 9512341 3 34 2452345 5353 67 22 42136357013412 42 5 65 64
and count how many times each digit appears.
examples/groovy/count_digits.groovy
counter = [0] * 10 fh = new File('examples/data/count_digits.txt') reader = fh.newReader() while ((line = reader.readLine()) != null) { for (c in line) { if (c in ' ') { continue } counter[ c as Integer ]++ } } for (i=0; i<10; i++) { println i + ' ' + counter[i] }
The expression in the first line creates a list of 10 values of 0.
Then we open the file for reading and create a reader that can read the file line-by-line.
Then we iterate over the lines one-by-one and use the for in construct to iterate over the characters of the string.
Inside the for loop first we check if this is the space charcter. In this solution we assume that the only unwanted character in the whole file is the space.
For every other character we use them as an integer to be the index in the counter list and increment the appropriate number.
At the end we have another for loop to iterate over all the digits and print the number of occurrences.
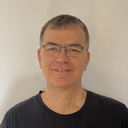
Published on 2018-06-03