Groovy: reading and writing files - appending content
In order to handle files Groovy has a class called File. Let's see some of the basic operations you can do with files.
examples/groovy/read_file.groovy
filename = 'examples/data/count_digits.txt' // read all the content of the file into a single string File fh1 = new File(filename) text = fh1.getText('UTF-8') // read all the lines into a list, each line is an element in the list File fh2 = new File(filename) def lines = fh2.readLines() for (line in lines) { // ... } File fh3 = new File(filename) LineNumberReader reader = fh3.newReader() while ((line = reader.readLine()) != null) { // ... }
Write a new file
This will remove the content of the old file and start a new file.
examples/groovy/write_file.groovy
File file = new File("out.txt") file.write "First line\n" file << "Second line\n" println file.text
This will append to the end of the file.
examples/groovy/append_file.groovy
File file = new File("out.txt") file.append("hello\n") println file.text
Write and Append
If we mix the calls to write and to append we'll get some strange results. For example the following code will only store "third" in the file.
examples/groovy/write_append_file.groovy
File file = new File("out.txt") file.write("first\n") file.append("second\n") file.write("third\n") println file.text
Don't do this!
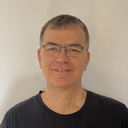
Published on 2018-06-03